
Vue Table With Image:Vue Table With Image is a Vue.js component used to display tabular data with images. It allows you to easily display and sort data in a table format while also including images in each row. This component is designed to be customizable and user-friendly, with options to adjust column widths, add filters, and paginate the data. It is particularly useful for displaying data such as product listings or user profiles, where images are an important aspect of the information being presented. Overall, Vue Table With Image is a versatile and convenient component for displaying tabular data with images in Vue.js applications.
What is the most efficient way to implement a Vue table component that includes an image for each row?
The code is a Vue table component that displays a list of users with their profile image, name, and email. It uses a v-for
directive to iterate over the users
data array and dynamically render a row for each user. The :key
binding is used to provide a unique identifier for each row.
For the profile image, it uses an img
element with the :src
binding to display the image URL from the user.profileImage
property. The alt
attribute provides alternative text for screen readers, and the width
attribute sets the image width.
Vue Table With Image Example
<div id="app">
<table class="table">
<thead>
<tr>
<th>Profile Image</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr v-for="(user, index) in users" :key="index">
<td><img :src="user.profileImage" alt="Profile Image" width="50"></td>
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
</tr>
</tbody>
</table>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
users: [
{
name: "John Doe",
email: "johndoe@example.com",
profileImage: "https://pixabay.com/get/g3ce20d761a08c657af2feb51c7492ae0ed3b24de16ad7835b7dca8d3e13991f6a12ca63433255db4d9a406a07da80768_640.jpg"
},
{
name: "Jane Doe",
email: "janedoe@example.com",
profileImage: "https://pixabay.com/get/g325ed0b5d83e018d5b7637a73d1ffc4a8bf742e8f76f487eed8c46dba9baecdadb9274d568e0a2d825ae5eef664fbbad82b1bb314fe6ad699f971ac322557dfe_640.jpg"
},
{
name: "Bob Smith",
email: "bobsmith@example.com",
profileImage: "https://pixabay.com/get/g6e7a962792b0987336743f1c4ec8d62aa3279d26c0b54516864f3ac72bd5d2970fbfade1770b5cf24aae85769e833776912ee3adaaf2f1dd65b3f751d06b79d0_640.jpg"
}
]
};
}
});
app.mount('#app');
</script>
Output of Vue Table with Image
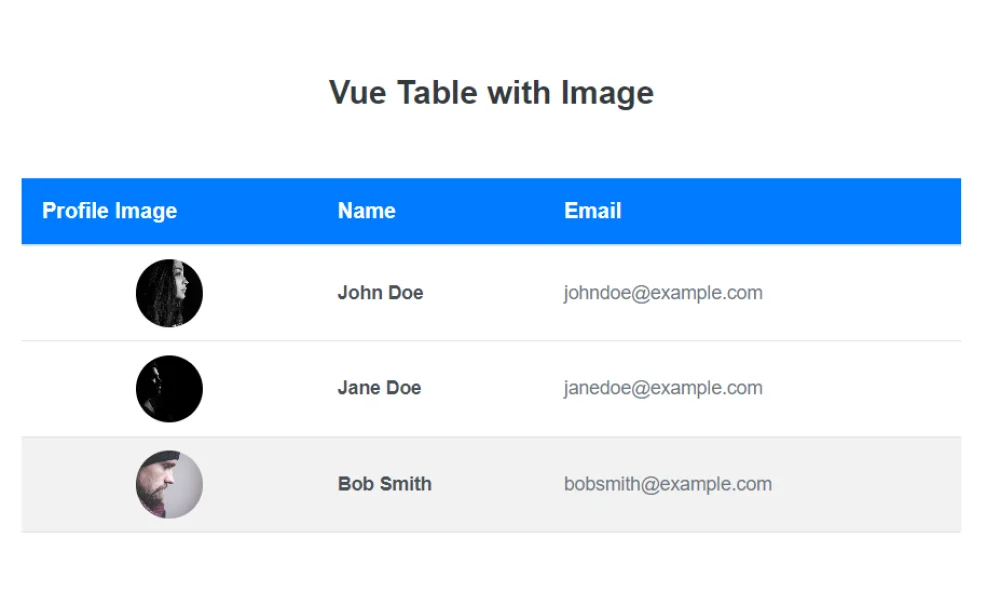